Os.walk takes care of the details, and on every pass of the loop, it gives us three things: dirName: The next directory it found. SubdirList: A list of sub-directories in the current directory. FileList: A list of files in the current directory. Let's say we have a directory tree that looks like this. Os.path module is sub module of OS module in Python used for common path name manipulation. Os.path.relpath method in Python is used to get a relative filepath to the given path either from the current working directory or from the given directory. Note: This method only computes the relative path. The existence of the given path or directory. File Names, Command Line Arguments, and Environment Variables¶ In Python, file names.
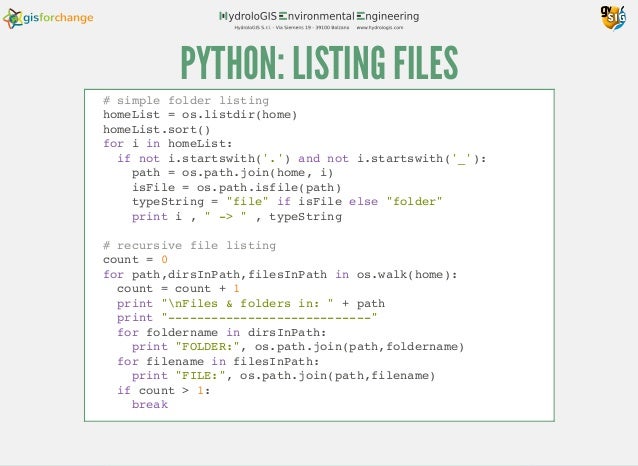
defwalk(top, topdown=True, onerror=None, followlinks=False): |
''Directory tree generator. |
For each directory in the directory tree rooted at top (including top |
itself, but excluding '.' and '..'), yields a 3-tuple |
dirpath, dirnames, filenames |
dirpath is a string, the path to the directory. dirnames is a list of |
the names of the subdirectories in dirpath (excluding '.' and '..'). |
filenames is a list of the names of the non-directory files in dirpath. |
Note that the names in the lists are just names, with no path components. |
To get a full path (which begins with top) to a file or directory in |
dirpath, do os.path.join(dirpath, name). |
If optional arg 'topdown' is true or not specified, the triple for a |
directory is generated before the triples for any of its subdirectories |
(directories are generated top down). If topdown is false, the triple |
for a directory is generated after the triples for all of its |
subdirectories (directories are generated bottom up). |
When topdown is true, the caller can modify the dirnames list in-place |
(e.g., via del or slice assignment), and walk will only recurse into the |
subdirectories whose names remain in dirnames; this can be used to prune |
the search, or to impose a specific order of visiting. Modifying |
dirnames when topdown is false is ineffective, since the directories in |
dirnames have already been generated by the time dirnames itself is |
generated. |
By default errors from the os.listdir() call are ignored. If |
optional arg 'onerror' is specified, it should be a function; it |
will be called with one argument, an os.error instance. It can |
report the error to continue with the walk, or raise the exception |
to abort the walk. Note that the filename is available as the |
filename attribute of the exception object. |
By default, os.walk does not follow symbolic links to subdirectories on |
systems that support them. In order to get this functionality, set the |
optional argument 'followlinks' to true. |
Caution: if you pass a relative pathname for top, don't change the |
current working directory between resumptions of walk. walk never |
changes the current directory, and assumes that the client doesn't |
either. |
Example: |
import os |
from os.path import join, getsize |
for root, dirs, files in os.walk('python/Lib/email'): |
print root, 'consumes', |
print sum([getsize(join(root, name)) for name in files]), |
print 'bytes in', len(files), 'non-directory files' |
if 'CVS' in dirs: |
dirs.remove('CVS') # don't visit CVS directories |
'' |
islink, join, isdir=path.islink, path.join, path.isdir |
# We may not have read permission for top, in which case we can't |
# get a list of the files the directory contains. os.path.walk |
# always suppressed the exception then, rather than blow up for a |
# minor reason when (say) a thousand readable directories are still |
# left to visit. That logic is copied here. |
try: |
# Note that listdir and error are globals in this module due |
# to earlier import-*. |
names=listdir(top) |
excepterror, err: |
ifonerrorisnotNone: |
onerror(err) |
return |
dirs, nondirs= [], [] |
fornameinnames: |
ifisdir(join(top, name)): |
dirs.append(name) |
else: |
nondirs.append(name) |
iftopdown: |
yieldtop, dirs, nondirs |
fornameindirs: |
new_path=join(top, name) |
iffollowlinksornotislink(new_path): |
forxinwalk(new_path, topdown, onerror, followlinks): |
yieldx |
ifnottopdown: |
yieldtop, dirs, nondirs |
In this article we will discuss different methods to generate a list of all files in directory tree.
Creating a list of files in directory and sub directories using os.listdir()
Python’s os module provides a function to get the list of files or folder in a directory i.e.
It returns a list of all the files and sub directories in the given path.
We need to call this recursively for sub directories to create a complete list of files in given directory tree i.e.
Call the above function to create a list of files in a directory tree i.e.
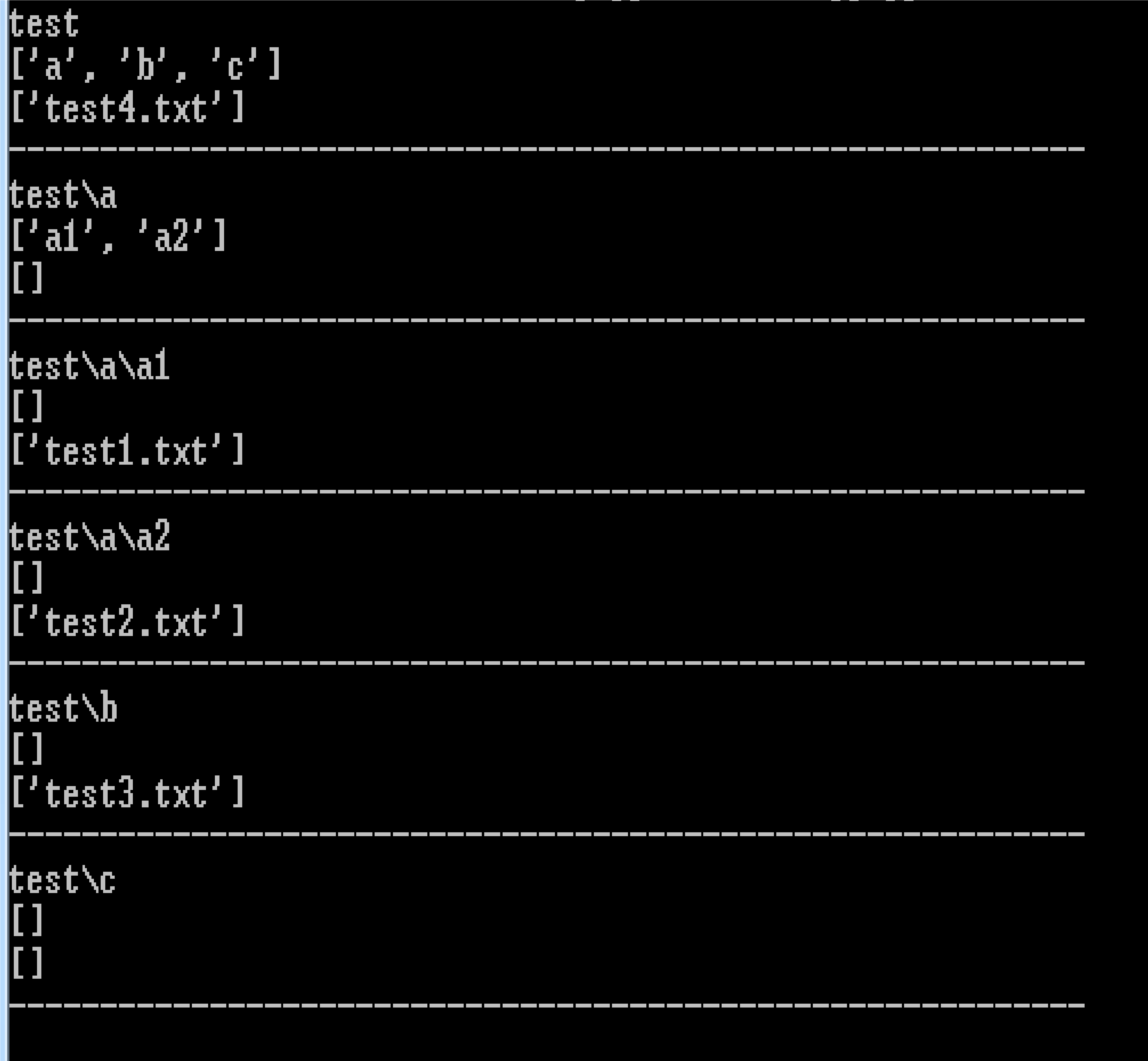
Creating a list of files in directory and sub directories using os.walk()

Walk The Path Os Srrow
Python’s os module provides a function to iterate over a directory tree i.e.
It iterates of the directory tree at give path and for each directory or sub directory it returns a tuple containing,
(<Dir Name> , <List of Sub Dirs> , <List of Files>.
Iterate over the directory tree and generate a list of all the files at given path,
Complete example is as follows,
Output:
Os.path.walk(path Visit Arg)
Join a list of 2000+ Programmers for latest Tips & Tutorials
Os Path Walk Python 3
Related Posts:
